In the process of fulfilling our requirements, there are instances when we require a popup for specific operations. To address this need, we utilize Visualforce pages and Apex controllers. Let’s commence with the steps to display a popup on Visualforce pages.
Navigate to Setup, then select Visualforce Pages, and click on “New.”
Next, insert the following code:
<apex:page controller="popupClass"> <apex:form> <apex:commandButton value="Show" action="{!showPopup}" rerender="tstpopup"/> <apex:outputPanel id="tstpopup"> <apex:outputPanel styleClass="popupBackground" layout="block" rendered="{!displayPopUp}"/> <apex:outputPanel styleClass="custPopup" layout="block" rendered="{!displayPopUp}"> <apex:commandButton value="Hide Pop up" action="{!closePopup}" rerender="tstpopup"/> </apex:outputPanel> </apex:outputPanel> </apex:form> <style type="text/css"> .custPopup{ background-color: white; border-width: 2px; border-style: solid; z-index: 9999; left: 50%; padding:11px; position: absolute; width: 600px; margin-left: -240px; top:100px; } .popupBackground{ background-color:black; opacity: 0.20; filter: alpha(opacity = 20); position: absolute; width: 100%; height: 100%; top: 0; left: 0; z-index: 9998; } </style> </apex:page>
Following are steps to create apex class:
Setup -> Apex Classes -> new
Put following code :
public class popupClass { public boolean displayPopup {get;set;} public void closePopup() { displayPopup = false; } public void showPopup() { displayPopup = true; } }
The following represents the output of the code mentioned above:
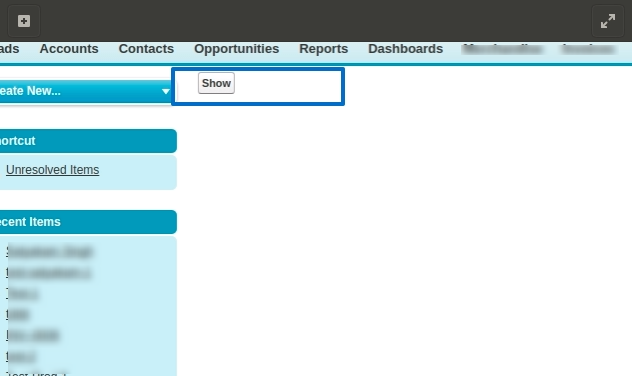
When you click on ‘Show’ button
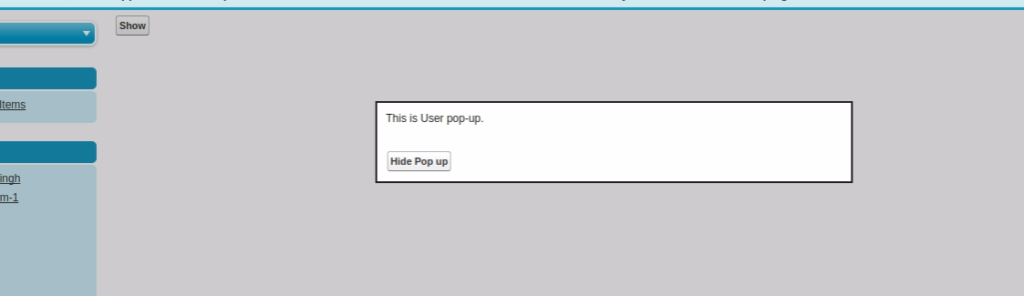