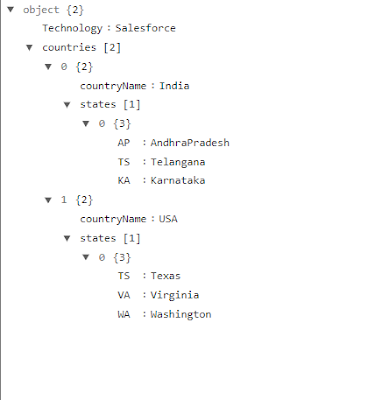
String data = '{"Technology":"Salesforce","countries":[{"countryName":"India","states":[{"AP":"AndhraPradesh","TS":"Telangana","KA":"Karnataka"}]},{"countryName":"USA","states":[{"TS":"Texas","VA":"Virginia","WA":"Washington"}]}]}'; Map<String, Object> mapData = (Map<String, Object>) JSON.deserializeUntyped(data); //get the Technology value String technVal = String.valueOf(mapData.get('Technology')); system.debug('==Technology value==='+technVal); //output: Salesforce //get the countries list List<Object> countriesList = (List<Object>) mapData.get('countries'); system.debug('==countriesList=='+countriesList); //output: ({countryName=India, states=({AP=AndhraPradesh, KA=Karnataka, TS=Telangana})}, {countryName=USA, states=({TS=Texas, VA=Virginia, WA=Washington})}) //get the first record from the countriesList system.debug('==First record=='+countriesList[0]); //output: {countryName=India, states=({AP=AndhraPradesh, KA=Karnataka, TS=Telangana})} //get the second record from the countriesList system.debug('==Second record=='+countriesList[1]); //output: {countryName=USA, states=({TS=Texas, VA=Virginia, WA=Washington})} //get the key and value of the first record Map<String, Object> firstRecord = (Map<String, Object>) countriesList[0]; String countryName = String.valueOf(firstRecord.get('countryName')); system.debug('==FirstRecord CountryName=='+countryName); //output: India //get the states value based on the key List<Object> statesList = (List<Object>) firstRecord.get('states'); Map<String, Object> mapstatesData = (Map<String, Object>) statesList[0]; String apStateVal = String.valueOf(mapstatesData.get('AP')); System.debug('==AP state value =='+apStateVal); //output: AndhraPradesh