Definition of a Trigger
An Apex trigger is a mechanism employed to execute specific actions during the process of saving records to the Salesforce database. Operating at the database level, it functions autonomously without the need for human intervention.
For instance, suppose a user enters an Opportunity Amount of $5000, and there’s another field named “Above $5000 Opportunity Checkbox” on the Opportunity object. With a trigger, you can manipulate the state of this checkbox—whether it should be true or false—based on the entered amount. We will delve into writing such a trigger later in this section.
Types of Apex Triggers
Triggers operate under various conditions:
- Before insert
- Before update
- Before delete
- After insert
- After update
- After delete
Where to craft your inaugural Apex Trigger.
You can create a Trigger in the Develop section of the Setup Menu, as depicted below.
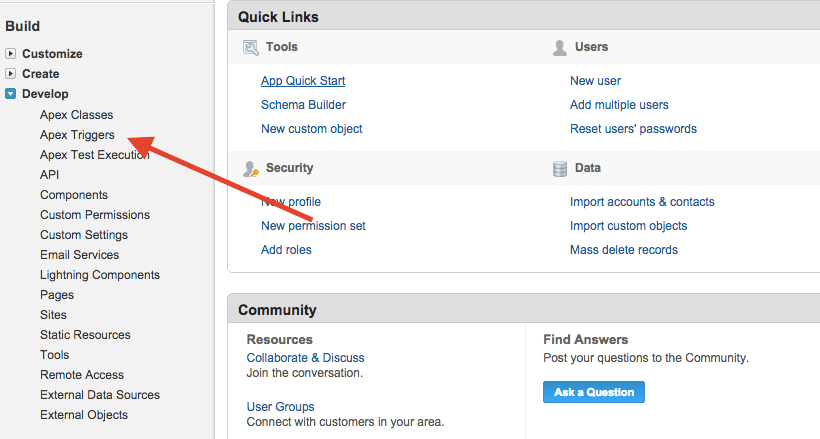
Trigger Context Variables
Various context variables are accessible within a trigger, enabling manipulation of records received during trigger execution.
Variable | Usage |
---|---|
isExecuting | Returns true if the current context for the Apex code is a trigger, not a Visualforce page, a Web service, or an executeanonymous() API call. |
isInsert | Returns true if this trigger was fired due to an insert operation, from the Salesforce user interface,Apex, or the API. |
isUpdate | Returns true if this trigger was fired due to an update operation, from the Salesforce user interface,Apex, or the API. |
isDelete | Returns true if this trigger was fired due to a delete operation, from the Salesforce user interface,Apex, or the API. |
isBefore | Returns true if this trigger was fired before any record was saved. |
isAfter | Returns true if this trigger was fired after all records were saved. |
isUndelete | Returns true if this trigger was fired after a record is recovered from the Recycle Bin (that is, after an undelete operation from the Salesforce user interface, Apex, or the API.) |
new | Returns a list of the new versions of the sObject records.Note that this sObject list is only available in insert and update triggers, and the records can only be modified in before triggers. |
newMap | A map of IDs to the new versions of the sObject records.Note that this map is only available in before update, after insert, and after updatetriggers. |
old | Returns a list of the old versions of the sObject records.Note that this sObject list is only available in update and delete triggers. |
oldMap | A map of IDs to the old versions of the sObject records.Note that this map is only available in update and delete triggers. |
size | The total number of records in a trigger invocation, both old and new. |
How to Create Your Initial Apex Trigger
Any trigger within Salesforce can be authored using the following syntax:
trigger triggername on objectname(event name)
For instance, if we intend to craft a trigger on the Opportunity object to update the Large_Opp__c
field, the code would resemble this:
Trigger opptrigger on opportunity(before insert) { //Loop Through All the records which are inside Trigger.new for(Opportunity opp : Trigger.new) { //logic to check value if(opp.amount>=5000) { //update value opp.Large_Opp__c = true; } } }
In the above trigger, you can observe that I’m modifying the value of the Large_Opp__c field on the Opportunity object before insertion. This demonstrates that we can adjust any value before it’s saved into the database. [Reference:]