Imagine you’re tasked with developing a Lightning component that exhibits records for a specific object. The selection of this object is facilitated through a dynamically generated picklist when the component is integrated into the Lightning page via the App Builder.
Create DesignComponentExample.cmp
<aura:component implements="force:appHostable,flexipage:availableForAllPageTypes,flexipage:availableForRecordHome,force:hasRecordId,force:lightningQuickAction" controller="DesignQueryUtils" access="global" > <aura:attribute name="objectName" type="String" default=""/> <aura:attribute name="title" type="String" default=""/> <aura:attribute name="description" type="String" default=""/> <aura:attribute name="recordsList" type="Object" /> <aura:attribute name="columns" type="list" /> <aura:handler name="init" value="{!this}" action="{!c.loadRecords}"/> <!-- Data Table here--> <div style="height:300px"> <b>{!v.title}</b> <lightning:datatable keyField="id" data="{! v.recordsList }" columns="{! v.columns }" hideCheckboxColumn="true"/> <div> {!v.description} </div> </div> </aura:component>
Generate DesignComponentExampleController.js.
({ loadRecords : function(component, event, helper) { component.set('v.columns', [ {label: 'Name', fieldName: 'Name', type: 'text'}, ]); var objName=component.get('v.objectName'); console.log(objName); var action=component.get("c.retrieveDetails"); action.setParams({ "sObjectName":objName }); action.setCallback(this,function(response){ var records = response.getReturnValue(); console.log(records); component.set("v.recordsList",records); }); $A.enqueueAction(action); } })
Develop DesignQueryUtils.apxc.
public class DesignQueryUtils { //To fetch records dynamically @AuraEnabled public static List<sObject> retrieveDetails(String sObjectName){ List<sObject> sObjectList = new List<sObject>(); String query ='SELECT Id,Name FROM '+ sObjectName +' LIMIT 10'; system.debug('query'+query); sObjectList = Database.query(query); return sObjectList; } }
Generate CustomDynamicPicklist.apxc.
global class CustomDynamicPicklist extends VisualEditor.DynamicPickList{ // dynamic picklist creator global override VisualEditor.DataRow getDefaultValue(){ VisualEditor.DataRow defaultValue = new VisualEditor.DataRow('account', 'Account'); return defaultValue; } global override VisualEditor.DynamicPickListRows getValues() { VisualEditor.DynamicPickListRows pickList = new VisualEditor.DynamicPickListRows(); List<VisualEditor.DataRow> dataRowsList = new List<VisualEditor.DataRow>(); for(Schema.sobjectType sobjectTypeName:schema.getGlobalDescribe().values()){ VisualEditor.DataRow rowObj = new VisualEditor.DataRow(sobjectTypeName.getDescribe().getLabel(),sobjectTypeName.getDescribe().getName()); dataRowsList.add(rowObj); } pickList.addAllRows(dataRowsList); return pickList; } }
Finally, within the design resource, we incorporate the Apex class.
Generate the DesignComponentExample.design design resource.
<design:component> <design:attribute name="title" label="Enter title here"/> <design:attribute name="description" label="Enter description here"/> <design:attribute name="objectName" label="specify object name" datasource="apex://CustomDynamicPickList"/> </design:component>
The output looks like :
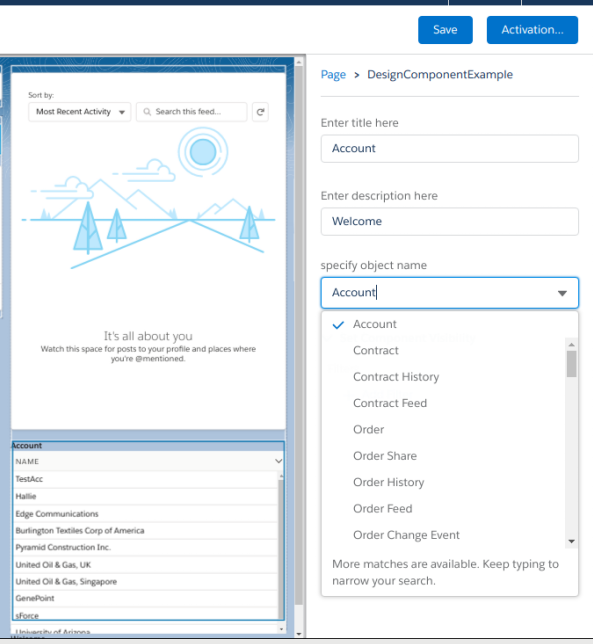
Dynamic picklist