Introduction:
Ensuring data security is imperative in any application to safeguard sensitive information. In Salesforce development, the management of confidential data, like customer details or financial information, is commonplace. While Salesforce provides inherent security features, there are instances where additional measures are necessary to fortify data protection. This blog post will guide you through the process of enhancing security by implementing Data Encryption and Decryption in Lightning Web Components, Salesforce’s contemporary UI framework.
Understanding Encryption and Decryption:
Encryption is like putting your data in a secret box. You use a key to lock the box (encrypt) so that only those with the right key can open it (decrypt) and see what’s inside. This helps keep your data safe from prying eyes, especially when it’s being sent over the internet or stored on servers.
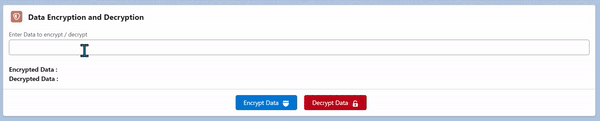
Implementing Custom Data Encryption in LWC:
For encrypting data in LWC, we will utilize a library known as CryptoJS, functioning as a toolkit for securing and releasing data. Below is a straightforward illustration of how to encrypt and decrypt data using CryptoJS:
<!-- @description : @author : Abhishek Verma @group : @last modified on : 02-14-2024 @last modified by : Abhishek Verma --> <template> <lightning-card variant="Narrow" title="Data Encryption and Decryption" icon-name="custom:custom91"> <div class="slds-p-horizontal_small"> <lightning-input type="text" label="Enter Data to encrypt / decrypt " value={Orgplaintext} onchange={handleChanges}></lightning-input> </div> <br> <div class="slds-p-horizontal_small"> <p> <b>Encrypted Data : </b>{encryptedData}</p> <p><b>Decrypted Data : </b>{decryptedData}</p> </div> <p slot="footer"> <lightning-button variant="brand" label="Encrypt Data" icon-name="utility:shield" icon-position="right" onclick={handleEncrypt} class="slds-m-left_x-small"></lightning-button> <lightning-button variant="destructive" label="Decrypt Data" onclick={handleDecrypt} icon-name="utility:unlock" class="slds-m-left_x-small" icon-position="right"></lightning-button> </p> </lightning-card> </template> import { LightningElement } from 'lwc'; import { loadScript } from 'lightning/platformResourceLoader'; import cryptoJS from '@salesforce/resourceUrl/cryptoJS'; export default class EncryptionComponent extends LightningElement { encryptedData; decryptedData; Orgplaintext; connectedCallback() { // Load CryptoJS script once the component is rendered Promise.all([ loadScript(this, cryptoJS) ]).then(() => { // CryptoJS script loaded successfully console.log('CryptoJS loaded'); }).catch(error => { // Error loading CryptoJS script console.error('Error loading CryptoJS:', error); }); } handleChanges(event){ this.Orgplaintext = event.target.value; } handleEncrypt() { console.log('this.Orgplaintext',this.Orgplaintext); const plaintext = this.Orgplaintext;//'Sensitive data to be encrypted'; const encryptionKey = 'SecretEncryptionKey'; const ciphertext = CryptoJS.AES.encrypt(plaintext, encryptionKey).toString(); this.encryptedData = ciphertext; console.log('Encrypted Data:', ciphertext); } handleDecrypt() { const ciphertext = this.encryptedData; // Encrypted data const decryptionKey = 'SecretEncryptionKey'; console.log('this.Orgplaintext',this.encryptedData); const bytes = CryptoJS.AES.decrypt(ciphertext, decryptionKey); const plaintext = bytes.toString(CryptoJS.enc.Utf8); console.log('Decrypted Data:', plaintext); this.decryptedData = plaintext; } }
In this code snippet, we input sensitive data (Orgplaintext), such as a password or credit card number, along with a secret key (encryptionKey). Subsequently, we employ CryptoJS to perform encryption and decryption utilizing the AES algorithm.
Closing Thoughts:
This aids in safeguarding sensitive information against unauthorized access. It’s crucial to ensure the secure storage of encryption keys and adhere to security best practices. Through appropriate implementation, we can bolster the overall security of our Salesforce applications, ensuring the safety of our users’ data.