Deciphering JSON Data in Apex, Salesforce’s unique programming language, is a routine task for developers. JSON (JavaScript Object Notation) stands as a lightweight data-interchange format, designed for easy comprehension by humans and machines alike. This blog post will explore several methodologies for parsing JSON data within Apex.
⓵ Employing the Inherent JSON.deserialize() Method The simplest and most direct approach to parse JSON data within Apex involves utilizing the built-in JSON.deserialize() method. This method necessitates a JSON string and a class type as inputs, ultimately generating an object of the designated class type. Consider a scenario where a JSON string represents a contact, and the aim is to deserialize it into a Contact object.
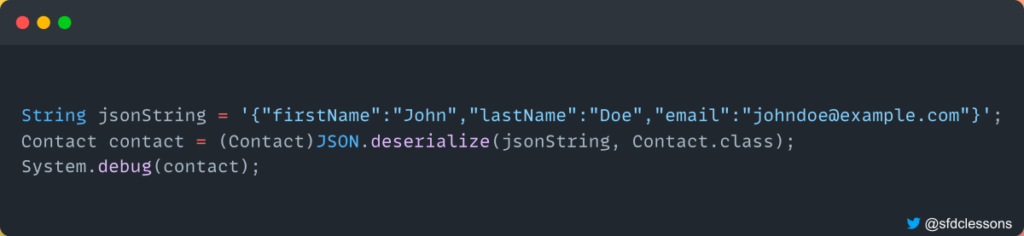
In this instance, the JSON string undergoes deserialization into a Contact object, and the debug statement will display the object’s attributes.
⓶ Employing the JSON.deserializeUntyped() Method When the JSON string is unknown during compilation or includes fields that don’t align with the Apex class fields, the JSON.deserializeUntyped() method becomes useful. This method yields a map of the JSON data, featuring keys as field names and their associated values.

In this instance, the JSON string undergoes deserialization into a map comprising String keys and Object values.
⓷ Utilizing the JSON.serialize() Method The JSON.serialize() method facilitates the transformation of an Apex object into a JSON string. It accepts an object as input and generates a JSON string representing that object.
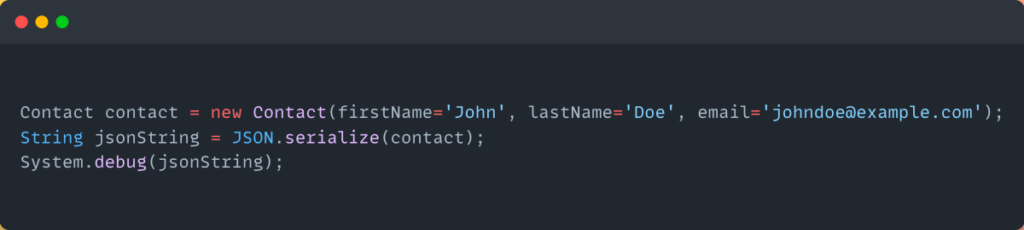
In this instance, the Contact object undergoes serialization into a JSON string, and the debug statement will display the resultant JSON string.
⓸ Apex JSON Parser: Apex offers a JSON.parser class, enabling the parsing of JSON data. This class includes methods like getCurrentValue() and nextToken() for navigating through the JSON data. It proves valuable when dealing with intricate JSON data that isn’t easily deserialized using the default Apex methods.
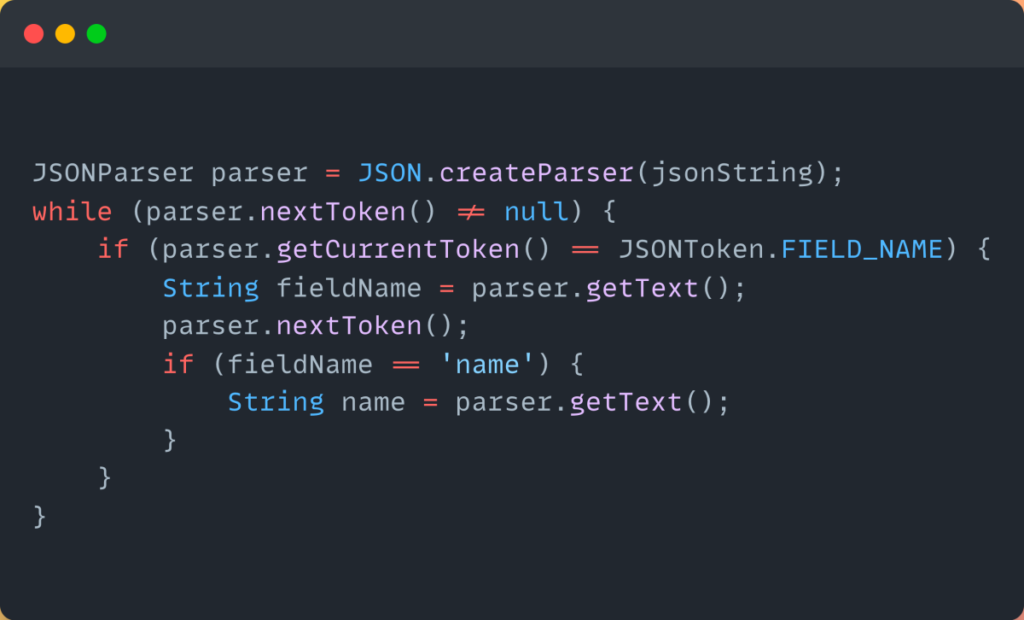
⓹ Employing External Libraries: Several third-party libraries exist for parsing JSON data in Apex. Well-known options encompass JSON2Apex, JSON.org, and JSON.simple. These libraries furnish supplementary functionalities and can prove beneficial when the default Apex methods fail to suffice.
JSON2Apex serves as an external library applicable for parsing JSON data within Apex. This library becomes valuable when the default Apex methods aren’t sufficient. It aids in generating Apex classes utilized for deserializing JSON strings into Apex objects. Here’s an example illustrating the usage of JSON2Apex to parse a JSON string:
Initially, utilize the JSON2Apex tool to generate the Apex class. Offer a sample JSON dataset, and the tool will generate the corresponding Apex class for you.
{ "name": "John Doe", "age": 35, "address": { "street": "123 Main St", "city": "Anytown", "state": "CA", "zip": "12345" }, "phoneNumbers": [ { "type": "home", "number": "555-555-1234" }, { "type": "work", "number": "555-555-5678" } ] }
The Apex class will be generated in this manner.
public class Example { public String name; public Integer age; public Address address; public List<PhoneNumbers> phoneNumbers; public class Address { public String street; public String city; public String state; public String zip; } public class PhoneNumbers { public String type; public String number; } }
Once the Apex class is generated, you can employ it to deserialize the JSON string. Here’s an example illustrating how to utilize the generated class for deserializing the JSON string into an Apex object:
String jsonString = '{ "name": "John Doe", "age": 35, "address": { "street": "123 Main St", "city": "Anytown", "state": "CA", "zip": "12345" }, "phoneNumbers": [ { "type": "home", "number": "555-555-1234" }, { "type": "work", "number": "555-555-5678" } ] }'; Example json = (Example)JSON.deserialize(jsonString, Example.class); System.debug(js
In this scenario, the JSON string undergoes deserialization into an Example object, and the debug statement will display the object’s attributes.
Please be aware that the class name may differ based on your chosen nomenclature during the class generation via JSON2Apex.
JSON2Apex stands as a valuable resource for developers contending with intricate JSON structures. It serves as a viable alternative when the default Apex methods fail to suffice. The tool enables seamless generation of Apex classes, simplifying the process of deserializing JSON strings into Apex objects, thereby saving valuable time and effort.
In summary, parsing JSON data in Apex stands as a pivotal task for developers, with multiple available approaches. While the built-in JSON.deserialize() method remains the simplest and most direct, alternatives such as JSON.deserializeUntyped() and JSON.serialize() are also viable. Third-party libraries offer supplementary functionalities as well.