MuleSoft has introduced the APIKit for GraphQL connector, which can be employed in conjunction with Anypoint Code Builder to create Mule applications.
What Is GraphQL?
GraphQL is an API query and manipulation language that enables consumers to fetch fields or data that are specifically relevant to their needs.
Use Case for GraphQL:
If we have a REST API that returns ‘n’ number of fields, but we encounter a scenario where a mobile app requires specific fields and a web app requires a different set of fields from the REST API, following the REST architecture would necessitate developing two separate REST APIs. However, this functionality can be achieved through a single endpoint in GraphQL. If the goal is to expose a unified endpoint capable of aggregating responses from multiple target systems, you can follow these steps to create a GraphQL application in Anypoint Code Builder:
- Create a GraphQL schema using the GraphQL editor. We’ve developed a GraphQL schema for the product and incorporated two queries: ‘productById’ and ‘products’.
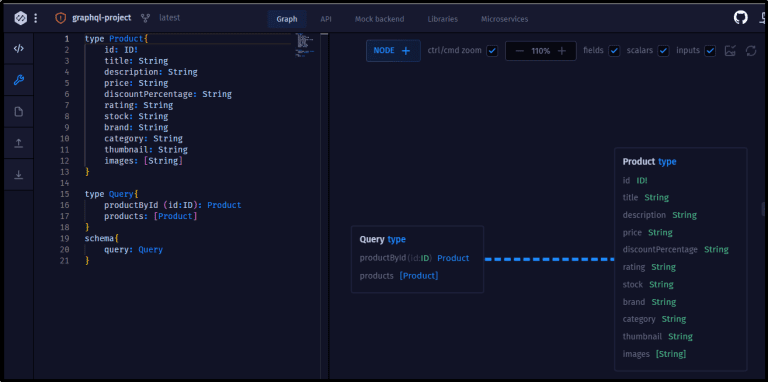
type Product{ id: ID! title: String description: String price: String discountPercentage: String rating: String stock: String brand: String category: String thumbnail: String images: [String] } type Query{ productById (id:ID): Product products: [Product] } schema{ query: Query }
Publish the Product GraphQL schema to Exchange as a GraphQL API asset. Furthermore, you have the option to implement the GraphQL API available in Exchange.
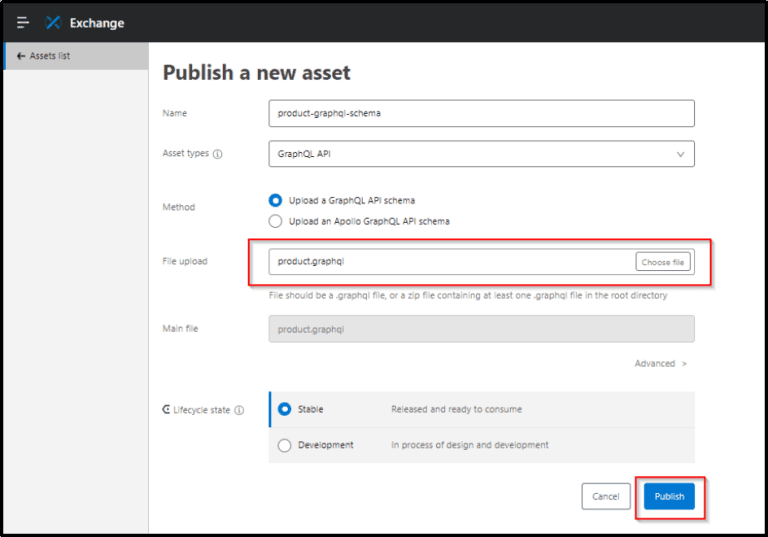
Open Anypoint Code Builder as a web-based integrated development environment (IDE) and select ‘Implement an API
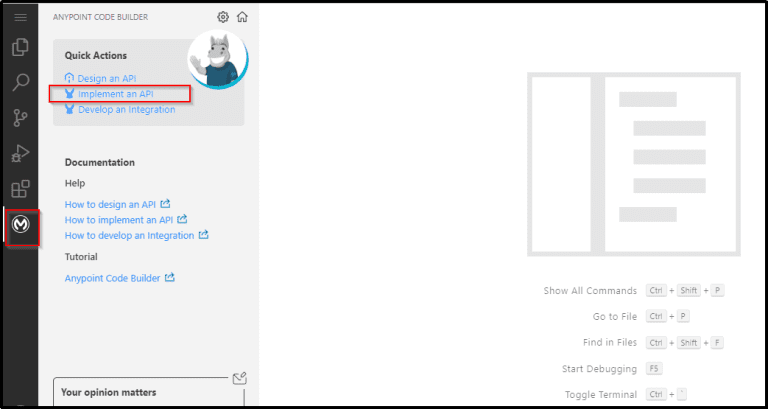
Configure the following fields and click ‘Create Project’:
- Project Name: Name of the Mule project
- Project Location: The directory where the project should be stored
- Search for an API specification published in Exchange to implement. Browse Exchange and select the GraphQL schema.
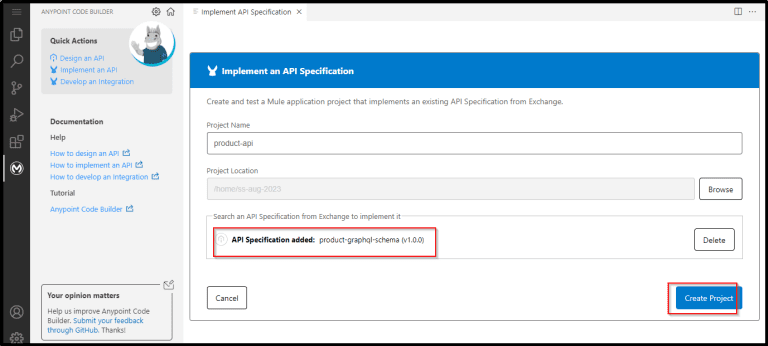
Anypoint Code Builder automatically generates Mule flows for each type defined in the Product GraphQL schema.
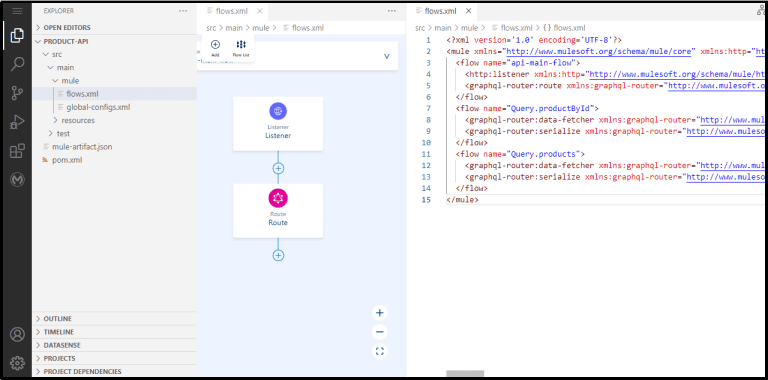
Integrate the logic to fetch product data from backend APIs into each flow. In our case, we have utilized a dummy JSON product API to retrieve product details.
Include a Request Configuration in the global-config.xml file.
<http:request-config name="Product API Config" doc:name="Product API Config" doc:id="af4dc2fc-0e8e-455c-8a7f-94b5a40e0c83" responseTimeout="120000"> <http:request-connection host="dummyjson.com" port="443" protocol="HTTPS" /> </http:request-config>
Completed Mule Flow Code
<?xml version='1.0' encoding='UTF-8'?> <mule xmlns="http://www.mulesoft.org/schema/mule/core" xmlns:doc="http://www.mulesoft.org/schema/mule/documentation" xmlns:http="http://www.mulesoft.org/schema/mule/http" xmlns:graphql-router="http://www.mulesoft.org/schema/mule/graphql-router" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:ee="http://www.mulesoft.org/schema/mule/ee/core" xsi:schemaLocation="http://www.mulesoft.org/schema/mule/core http://www.mulesoft.org/schema/mule/core/current/mule.xsd http://www.mulesoft.org/schema/mule/http http://www.mulesoft.org/schema/mule/http/current/mule-http.xsd http://www.mulesoft.org/schema/mule/graphql-router http://www.mulesoft.org/schema/mule/graphql-router/current/mule-graphql-router.xsd http://www.mulesoft.org/schema/mule/ee/core http://www.mulesoft.org/schema/mule/ee/core/current/mule-ee.xsd"> <flow name="api-main-flow"> <http:listener xmlns:http="http://www.mulesoft.org/schema/mule/http" config-ref="http-listener-config" path="${http.listener.path}" /> <graphql-router:route xmlns:graphql-router="http://www.mulesoft.org/schema/mule/graphql-router" config-ref="api-router-config" /> </flow> <flow name="Query.productById"> <graphql-router:data-fetcher xmlns:graphql-router="http://www.mulesoft.org/schema/mule/graphql-router" config-ref="api-router-config" objectTypeName="Query" fieldName="productById" /> <http:request method="GET" doc:name="Request" doc:id="9007ba6a-8019-438a-9415-892c8294a14d" config-ref="Product API Config" path="/products/{productid}"> <http:uri-params> <![CDATA[#[%dw 2.0 output application/json --- { "productid": attributes.arguments.id }]]]> </http:uri-params> </http:request> <graphql-router:serialize xmlns:graphql-router="http://www.mulesoft.org/schema/mule/graphql-router" config-ref="api-router-config" objectTypeName="Query" fieldName="productById" /> </flow> <flow name="Query.products"> <graphql-router:data-fetcher xmlns:graphql-router="http://www.mulesoft.org/schema/mule/graphql-router" config-ref="api-router-config" objectTypeName="Query" fieldName="products" /> <http:request method="GET" doc:name="Request" doc:id="8007ba6a-8019-438a-9415-892c8294a14d" config-ref="Product API Config" path="/products"></http:request> <ee:transform doc:name="Transform Message" doc:id="lvtqis"> <ee:message> <ee:set-payload doc:name="Set payload" doc:id="wxopxb"> <![CDATA[%dw 2.0 output application/json --- payload.products ]]> </ee:set-payload> </ee:message> </ee:transform> <graphql-router:serialize xmlns:graphql-router="http://www.mulesoft.org/schema/mule/graphql-router" config-ref="api-router-config" objectTypeName="Query" fieldName="products" /> </flow> </mule>
Testing the GraphQL Implementation:
Go to the Run and Debug view, and then click the run button to deploy the Mule application.
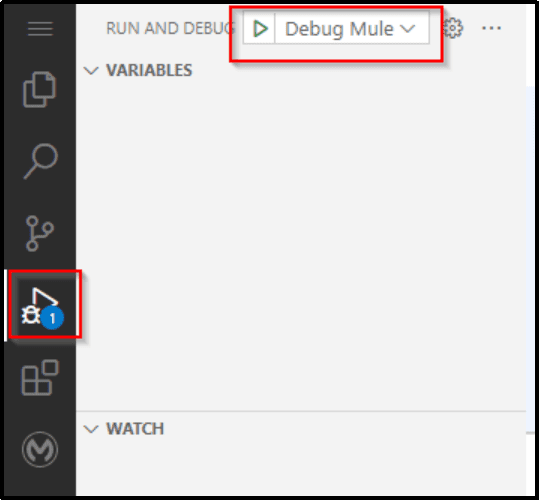
To test your Mule application from a REST client like Postman, you need to include a ‘proxysession’ cookie when making a call to the GraphQL service. You can obtain this value using Developer Tools. Please copy both the cookie name and its associated value.
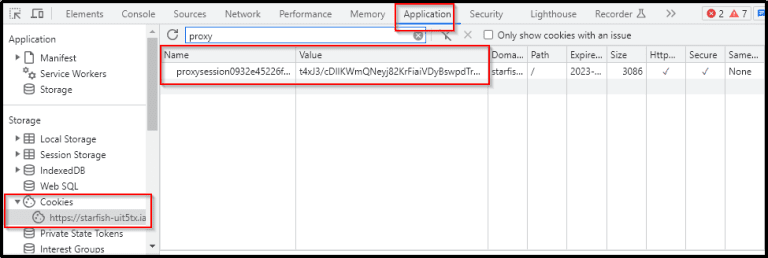
Visit the PORTS tab and retrieve the local address for port 8081.

In Postman, start a POST request using the local address and add the /GraphQL path. Be sure to include the ‘proxysession’ cookie, using the cookie value in the format ‘name=value’.

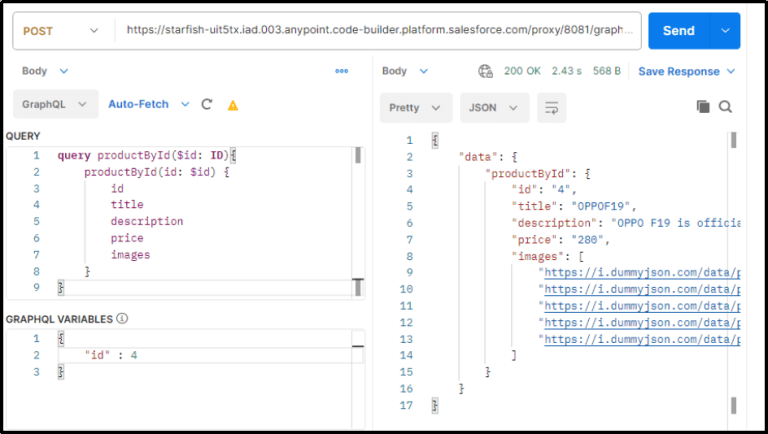
productById Query – Snapshot 1
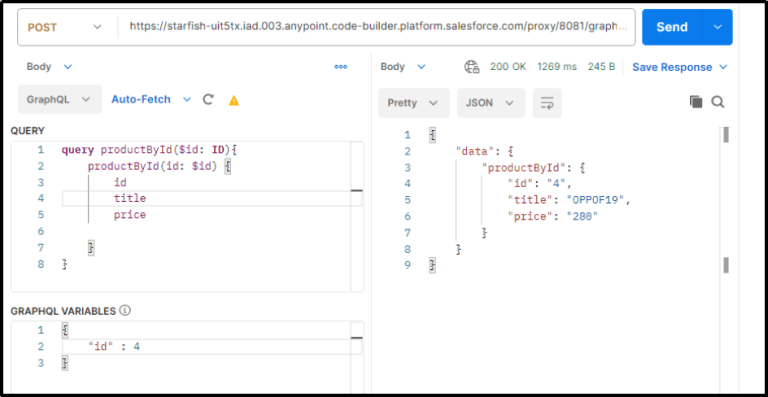
productById Query – Snapshot 2
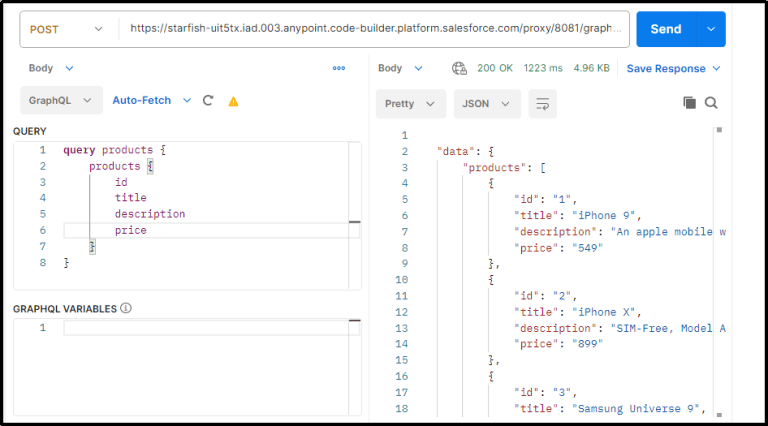
Products Query – Snapshot
Please be aware that using Anypoint Code Builder in a production environment is not recommended, as it is currently in beta release. We hope you found this blog post informative and enjoyable.