Creating a Salesforce Record with Lightning Web Components and Apex Class
Utilize the lightning-input
element to capture user input for Account Name, Phone, Rating, and Industry. Incorporate a lightning-button
to invoke the JavaScript controller method responsible for creating the record. Implement an onchange
handler for each lightning-input
tag to retrieve the modified values in the JavaScript controller.
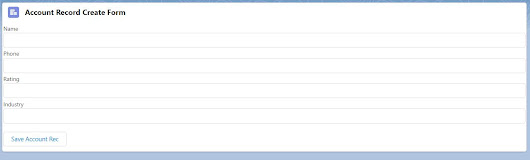
1. Create HTML Templete
<template> <lightning-card title="Account Record Create Form" icon-name="standard:account"> <div class="slds-var-p-around_x-small"> <lightning-input value={recAccount.Name} label="Account Name" onchange={handelNamechange}></lightning-input> <lightning-input value={recAccount.Phone} label="Phone" onchange={handelPhonechange}></lightning-input> <lightning-input value={recAccount.Rating} label="Rating" onchange={handelRatingchange}></lightning-input> <lightning-input value={recAccount.Industry} label="Industry" onchange={handelinduschange}></lightning-input> <br/> <lightning-button label="Save Account Rec" onclick={createAccRec} variant="brand"></lightning-button> </div> </lightning-card> </template>
2. Create Javascript File.
import { LightningElement, track } from 'lwc'; import NAME_FIELD from '@salesforce/schema/Account.Name'; import PHONE_FIELD from '@salesforce/schema/Account.Phone'; import RATING_FIELD from '@salesforce/schema/Account.Rating'; import INDUSTRY_FIELD from '@salesforce/schema/Account.Industry'; import accountRecMethod from '@salesforce/apex/AccountRecHelper.accountRecMethod'; import { ShowToastEvent } from 'lightning/platformShowToastEvent'; export default class AccountRecCreation extends LightningElement { @track name = NAME_FIELD; @track phone = PHONE_FIELD; @track rating = RATING_FIELD; @track industry = INDUSTRY_FIELD; recAccount = { Name : this.name, Phone : this.phone, Rating : this.rating, Industry: this.industry } handelNamechange(event){ this.recAccount.Name = event.target.value; //console.log("name",this.recAccount.Name); } handelPhonechange(event){ this.recAccount.Phone = event.target.value; // console.log("phone",this.recAccount.Phone); } handelRatingchange(event){ this.recAccount.Rating = event.target.value; // console.log("email",this.recAccount.Rating); } handelinduschange(event){ this.recAccount.Industry = event.target.value; //console.log("industry",this.recAccount.Industry); } createAccRec() { debugger; accountRecMethod({ accRec : this.recAccount }) .then(result => { this.message = result; this.error = undefined; if(this.message !== undefined) { this.recAccount.Name = ''; this.recAccount.Industry = ''; this.recAccount.Phone = ''; this.recAccount.Rating= ''; this.dispatchEvent( new ShowToastEvent({ title: 'Success', message: 'Account created', variant: 'success', }), ); } console.log(JSON.stringify(result)); console.log("result", this.message); }) .catch(error => { this.message = undefined; this.error = error; this.dispatchEvent( new ShowToastEvent({ title: 'Error creating record', message: error.body.message, variant: 'error', }), ); console.log("error", JSON.stringify(this.error)); }); } }
3. Create Apex Class
public with sharing class AccountRecHelper { @AuraEnabled public static Account accountRecMethod(Account accRec){ try { insert accRec; return accRec; } catch (Exception e) { throw new AuraHandledException(e.getMessage()); } } }