Possessing fundamental familiarity with the Salesforce Apex programming language is a valuable asset for any Salesforce administrator. This proficiency enables administrators to gain a deeper comprehension of application behavior, facilitating the ability to elucidate underlying logic to users and troubleshoot issues independently, without relying on a Salesforce developer for assistance.
To assist Salesforce administrators in delving into Apex coding, I suggest creating a Hello World program. In this guide, I will guide you through the process, allowing you to grasp essential programming concepts such as variables, primitives, conditionals, and methods.
Utilizing the Developer Console
Before we embark on coding our Hello World program, it’s essential to understand how to run it. The Salesforce platform offers various methods for executing Apex coding and programming, with one of the simplest being through the Developer Console for running code in an anonymous block. Let’s initiate this process by opening the console.
Ensure that your profile has the “Author Apex” permission activated.
- Click on the gear icon and choose Developer Console. If you’re in classic mode, click on your name at the top right and then select Developer Console.
- Once the Developer Console is loaded, click on “Debug” in the menu and choose “Open Execute Anonymous Window.” Alternatively, you can use the shortcut CTRL+E.
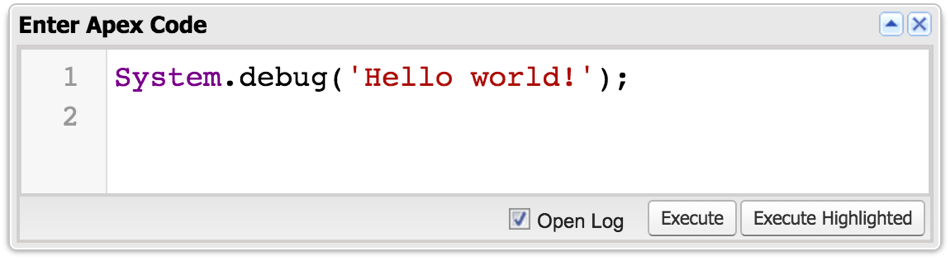
Image 1 – Execute Anonymous Window
Type the following line of code:
System.debug('Hello world!');
Enable the “Open Log” option. Click on Execute. After the Execution Log is loaded, select the “Debug Only” checkbox. You should now observe your “Hello, World!” message. Congratulations, you have successfully authored your initial Salesforce Apex program!
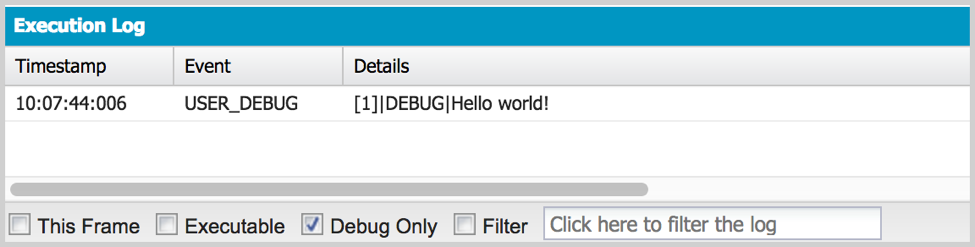
Image 2 – Execution Log
Variables within the Salesforce Apex Programming Language
Moving on to our next step, let’s delve into something more engaging. We’ll incorporate a variable, assign the value “Hello World!” to it, and subsequently display the variable’s value in the debug log.
Open the Execute Anonymous Window through the debug menu or use the shortcut CTRL+E. Adjust your code to resemble the following:
String message = 'Hello world!'; System.debug(message);
Click on Execute.
After the Execution Log is loaded, select the “Debug Only” checkbox. Observe that the output remains the same as the previous code execution. Line 1 serves a dual purpose—firstly, it declares the variable, indicating to Apex the creation of a new variable of type String. Secondly, it assigns the value “Hello, World!” to the variable.An intriguing characteristic of variables is their mutability, allowing us to modify their content after creation. Let’s experiment with this by updating the code and adding an additional line.
String message = 'Hello world!'; message = 'Hi there!'; System.debug(message);
Overriding Your Values
Execute the code again by following the same steps as before. This time, you will observe “Hi there!” instead of “Hello, World!” Let’s analyze what just occurred: In line 1, we established a variable named “message” and assigned the value “Hello, World!” to it. Subsequently, in line 2, we assigned a new value, “Hi there,” effectively replacing the previous one through the process of overwriting.
The Salesforce Apex programming language allows you to conduct operations on variables. In this scenario, as we are working with Strings, various operations such as concatenation (joining two or more strings) or changing the casing to all uppercase can be performed.
String greeting = 'Hello'; String who = 'world'; who = who.toUpperCase(); String message = greeting + ' ' + who + '!'; System.debug(message);
Upon running this code, you’ll observe a result similar to the initial example. This is due to the concatenation of a variable named “greeting,” encompassing the word “Hello,” a space, the “who” variable containing “WORLD,” and the exclamation point symbol. The combination of these elements constructs the phrase “Hello WORLD!”
If you’re curious about why the word “WORLD” is entirely in uppercase, it’s attributed to line 3, where the toUpperCase() function is invoked. This function returns the same string but with all its letters capitalized.
Fundamental Data Types in the Salesforce Apex Programming Language
Up to this point, we’ve exclusively employed String-type variables in our illustrations. While String variables enable the storage of collections of characters, numbers, or symbols, there are additional types available for diverse purposes. For instance, Integer is suitable for storing whole numbers, while Double is employed for numbers with decimals. The Salesforce Apex programming language encompasses a total of 12 primitive types, and custom types can be fashioned by crafting your own classes.
Catalog of Fundamental Types in Salesforce Apex
Blob | Binary Data |
Boolean | The values true or false |
Date | A calendar date |
Datetime | A calendar date and time |
Decimal | A number that includes a decimal point |
Double | A double precision floating point number |
ID | A 15 or 18-digit Salesforce ID |
Integer | A whole number — between -2,147,483,648 and 2,147,483,647 |
Long | A large whole number — between -263 and 263-1 |
Object | A generic type that can store a value of any other type |
String | Any set of characters — surrounded by single quotes |
Time | A time |
Conditional Statements in the Salesforce Apex Programming Language
To enable your code to make decisions and exhibit distinct behavior contingent on variable values or user input, employing a conditional statement is necessary. The most prevalent type of conditional statement is the if-else statement, closely resembling the IF() function in Salesforce formulas. Analogous to its formula equivalent, it comprises three components: the logical test, the true block, and optionally, the false block. If the logical test assesses as true, the true block is executed; otherwise, the false/else block is executed. A code block refers to one or more lines of code enclosed within curly brackets.
Boolean formal = true; String who = 'world'; String greeting; if (formal == true) { greeting = 'Hello'; } else { greeting = 'Hi'; } String message = greeting + ' ' + who; System.debug(message);
Upon executing this code, you’ll observe an output of “Hello, world.” The logical test was validating whether the variable “formal” equaled true. Consequently, it executed the first code block (line 5), assigning the variable “greeting” to “Hello.” If the test had proven false, it would have triggered the false/else block (line 7), resulting in the variable “greeting” being set to “Hi.” Feel free to run it again, this time setting the variable “formal” to false on line 1.
Creating Functions in the Salesforce Apex Programming Language
As a Salesforce administrator, you likely have a grasp of the concept of methods, which closely resemble functions in validation rules and formula fields. In our examples, we’ve already employed two methods: System.debug() and String.toUpperCase(). You’re already acquainted with how to invoke them. Now, let’s delve into a more detailed examination.
Functions in Salesforce Apex
Methods embody a section of code that can be invoked or called from elsewhere. They serve various purposes but are primarily utilized for two main objectives. Firstly, methods provide a means to circumvent redundancy by writing code once and invoking it multiple times as required. Secondly, methods enhance code cleanliness and readability by facilitating the abstraction of specific sections.
Let’s enhance our Hello World program by relocating the greeting selection logic into a method, which we’ll name getGreeting().
Boolean formal = true; String who = 'world'; String greeting = getGreeting(formal); String message = greeting + ' ' + who; System.debug(message); private String getGreeting(Boolean formal) { if (formal == true) { return 'Hello'; } else { return 'Hi'; } }
Now, when you wish to quickly review the code, a cursory glance at the first 5 lines should provide a high-level understanding of its functionality. If you’re interested in the specifics of how the greeting is selected, you can examine lines 8 through 12 inside the method. This approach enhances the code’s readability, making it more accessible for others to comprehend or even for yourself in the future when you need to refresh your memory on the logic.
This latest version of the code will produce “Hello, world” just like the preceding versions, but the sequence in which the lines are executed varies slightly. Initially, lines 1 and 2 will run. Upon reaching line 3, to obtain the value for storing in “greeting,” lines 6, 7, and 8 will execute, and getGreeting() will return “Hello.” Subsequently, execution proceeds to line 4, where “Hello, world” is stored in the “message.” Finally, line 5 is executed, outputting the value of the message. At this point, the code concludes its execution and terminates.
Additional Resources for Exploring the Salesforce Apex Programming Language
Congratulations on completing this tutorial! At this point, you should possess the ability to interpret and grasp the essence of the code within your organization, even if the exact function of each line isn’t fully understood. Furthermore, you should feel confident in making basic modifications to existing code under the guidance of a more experienced developer.
If you found the process of creating your first program enjoyable and wish to delve deeper into the Apex programming language, consider exploring these excellent resources: