Custom Metadata Offers excellent flexibility to administrators and developers for crafting dynamic functionalities. In the context of Lightning Web Components, a common query is how custom metadata records can be efficiently accessed. The answer is that there is no need for an Apex call to retrieve your custom metadata records in Lightning Web Components.
Obtaining Custom Metadata in Lightning Web Components without Using Apex
Utilize the wire service and the getRecord wire adapter to retrieve record data in a Lightning Web Component. Let’s create a component named “lifeCycle” to obtain the custom metadata record without invoking an Apex method and display the value on the UI. Begin by creating a custom metadata in the developer org with the API name “Validation_Control__mdt,” which includes a custom field with the API name “isActive__c.
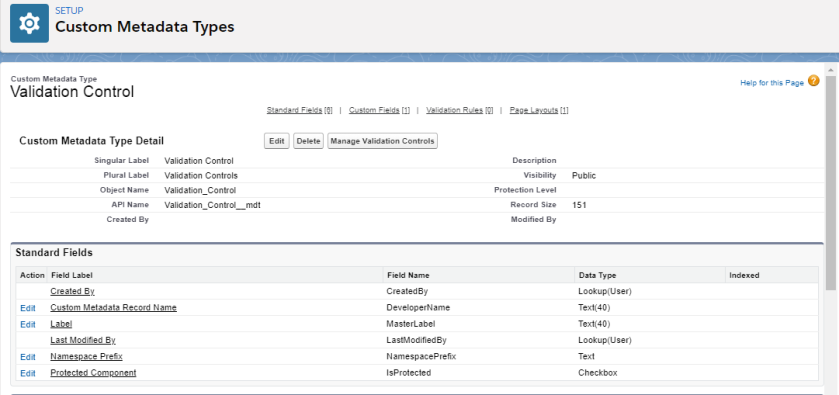
After creating the custom metadata object, a corresponding record has been generated. In the component’s JavaScript file, I have manually set the record ID for retrieval. Alternatively, you can dynamically pass the ID.
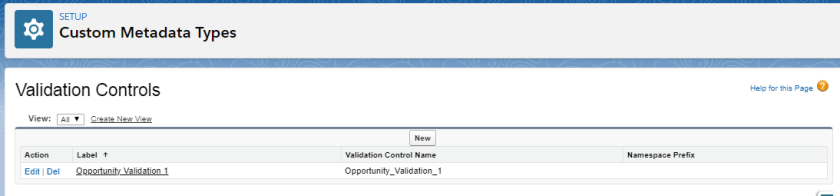
lifeCycle.html
<template> <lightning-card title="My Custom Metadata" icon-name="standard:contact"> <template if:true={metadatarecord.data}> <div class="slds-m-around_medium"> <p>{label}</p> <p>{developername}</p> <p>{active}</p> <p>{language}</p> </div> </template> </lightning-card> </template>
lifeCycle.js
First, the code imports the getRecord
wire adapter from the lightning/uiRecordApi
module, which is built on Lightning Data Service. Then it defines the fields to pass to the wire adapter.
The @wire
decorator tells getRecord
to get the values of the specified fields on the record with the specified $recordId
. The $
means that the value is passed dynamically. When the value changes, the wire service provisions data and the component rerenders.The data is provisioned to the data
and error
objects of the property decorated with @wire
.
import { LightningElement, wire } from 'lwc'; import { getRecord } from 'lightning/uiRecordApi'; const FIELDS = [ 'Validation_Control__mdt.MasterLabel', 'Validation_Control__mdt.DeveloperName', 'Validation_Control__mdt.isActive__c', 'Validation_Control__mdt.Language', ]; export default class LifeCycle extends LightningElement { //Id is hardcoded for demo purpose. You can pass a dynamic id here recordId='m00B00000001hihIAA'; @wire(getRecord, { recordId: '$recordId', fields: FIELDS }) metadatarecord; get label() { return this.metadatarecord.data.fields.MasterLabel.value; } get developername() { return this.metadatarecord.data.fields.DeveloperName.value; } get active() { return this.metadatarecord.data.fields.isActive__c.value; } get language() { return this.metadatarecord.data.fields.Language.value; } }
lifeCycle.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata"> <apiVersion>47.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__AppPage</target> <target>lightning__RecordPage</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
Demo