Have you encountered CORS problems when embedding Web-to-Lead or Web-to-Case forms on your website?
Cross-Origin Resource Sharing
Cross-Origin Resource Sharing (CORS) involves an HTTP header mechanism enabling a server to specify origins (domains, schemes, or ports) other than its own from which a browser can load resources. CORS errors arise when a server doesn’t provide the necessary HTTP headers as per the CORS standard. To address a CORS error in an API Gateway REST API or HTTP API, reconfiguring the API to adhere to the CORS standard is essential.
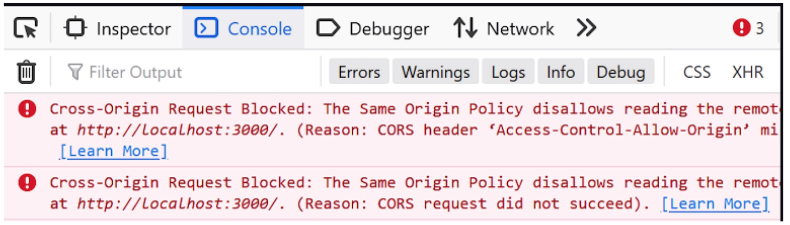
Solution
Here’s an alternative approach utilizing an HTML form and handling its processing via JavaScript.
<script type="text/javascript"> // Case Data preparation var postData = { oid: '00D0b000000xxxx', //Put your ORGID here name:'John Smith', company:'ACME', email:'yyy@customercompany.com', origin:'Web', subject:'Web2Case using the custom API', description:'This is an automated transparent Case sent using our custom API.' } // Send data to Salesforce webToCase(postData); // Web to Case function function webToCase(fields) { var customHiddenIframeName='JLA_API'; if(!document.getElementById(customHiddenIframeName)){ var theiFrame=document.createElement("iframe"); theiFrame.id=customHiddenIframeName; theiFrame.name=customHiddenIframeName; theiFrame.src='about:blank'; theiFrame.style.display='none'; document.body.appendChild(theiFrame); } fields['retURL']='http://127.0.0.1';//dummy URL var form = document.createElement("form"); form.method = "POST"; form.action = "https://webto.salesforce.com/servlet/servlet.WebToCase?encoding=UTF-8"; form.setAttribute("target", customHiddenIframeName); for (var fieldName in fields) { var theInput = document.createElement("input"); theInput.name=fieldName; theInput.value=fields[fieldName]; theInput.setAttribute("type", "hidden"); form.appendChild(theInput); } document.body.appendChild(form); form.submit(); } </script>
<script type="text/javascript"> // Lead Data preparation var postData = { oid: '00D0b000000xxxx', //Put your ORGID here first_name: 'M Hamza', last_name: 'Siddiqui', email: 'code@yopmail.com', phone: '1112223333', } // Send data to Salesforce webToLead(postData); // Web to lead function function webToLead(fields) { var customHiddenIframeName='JLA_API'; if(!document.getElementById(customHiddenIframeName)){ var theiFrame=document.createElement("iframe"); theiFrame.id=customHiddenIframeName; theiFrame.name=customHiddenIframeName; theiFrame.src='about:blank'; theiFrame.style.display='none'; document.body.appendChild(theiFrame); } fields['retURL']='http://127.0.0.1';//dummy URL var form = document.createElement("form"); form.method = "POST"; form.action = "https://webto.salesforce.com/servlet/servlet.WebToLead?encoding=UTF-8"; form.setAttribute("target", customHiddenIframeName); for (var fieldName in fields) { var theInput = document.createElement("input"); theInput.name=fieldName; theInput.value=fields[fieldName]; theInput.setAttribute("type", "hidden"); form.appendChild(theInput); } document.body.appendChild(form); form.submit(); } </script>
Enjoy your coding!