To iterate through a list, we employ two directives:
- for:each
- Iterator
When utilizing for:each or Iterator, it’s crucial to include the key directive on the iterated element. The key attribute provides a unique identifier for each item. It’s important to note that iteration is impossible without the key attribute.
The framework, upon list modification, utilizes the key to selectively re-render only the item that underwent changes. This mechanism serves as a performance optimization and isn’t visibly reflected in the DOM during runtime.
for:each
When employing the for:each directive, utilize for:item=”currentItem” to access the present item. Assign a key to the initial element within the nested template using the key={uniqueId} directive. Optionally, use for:index=”index” to access the current item’s index.
Example: HelloForEach.html
<template> <lightning-card title="HelloForEach" icon-name="custom:custom14"> <ul class="slds-m-around_medium"> <template for:each={contacts} for:item="contact"> <li key={contact.Id}> {contact.Name}, {contact.Title} </li> </template> </ul> </lightning-card> </template>
HelloForEach.js
import { LightningElement } from 'lwc'; export default class HelloForEach extends LightningElement { contacts = [ { Id: 1, Name: 'Amy Taylor', Title: 'VP of Engineering', }, { Id: 2, Name: 'Michael Jones', Title: 'VP of Sales', }, { Id: 3, Name: 'Jennifer Wu', Title: 'CEO', }, ]; }
Output:
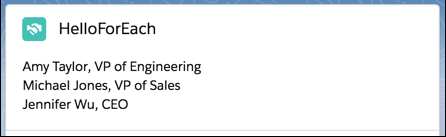
Another Practical Example:
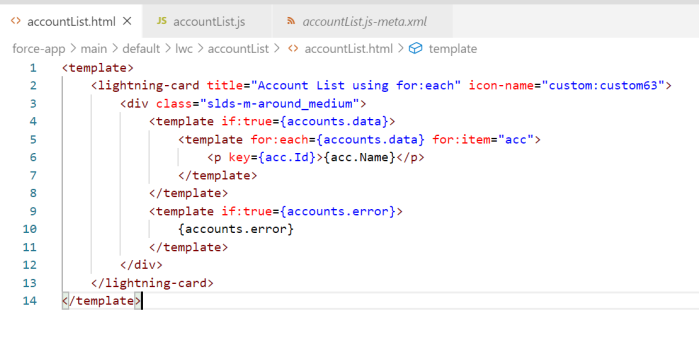
AccountList.hmtl
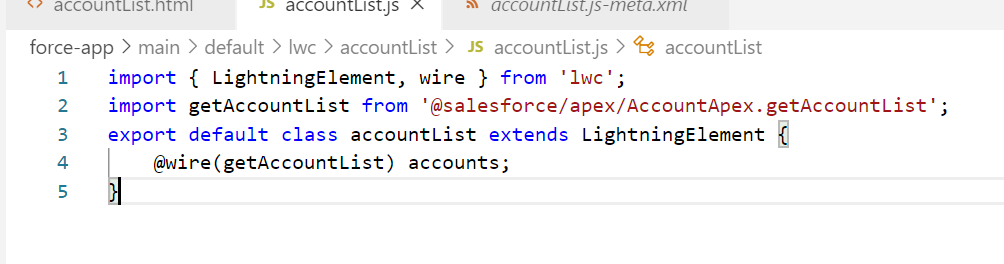
AccountList.js
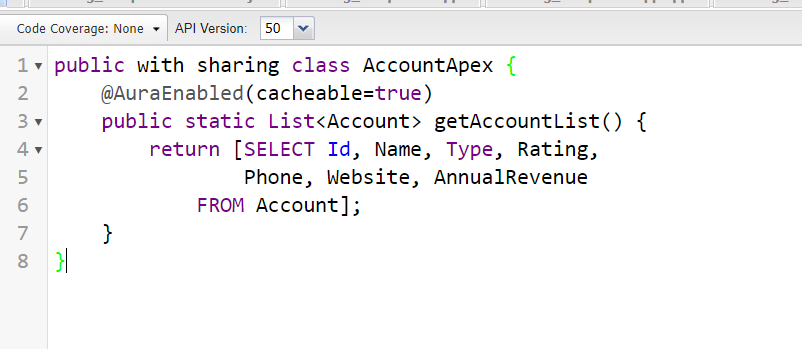
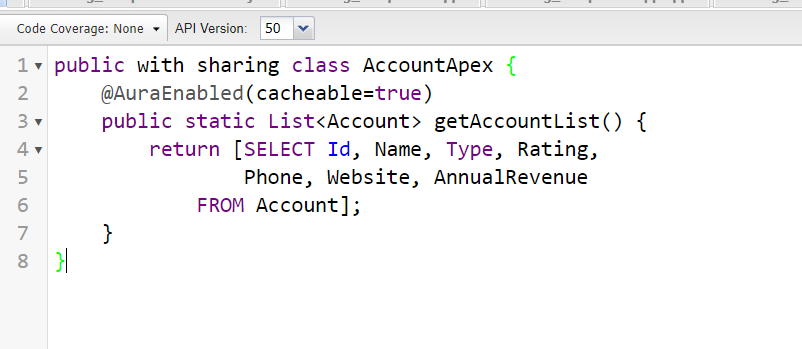
AccountApex.cls
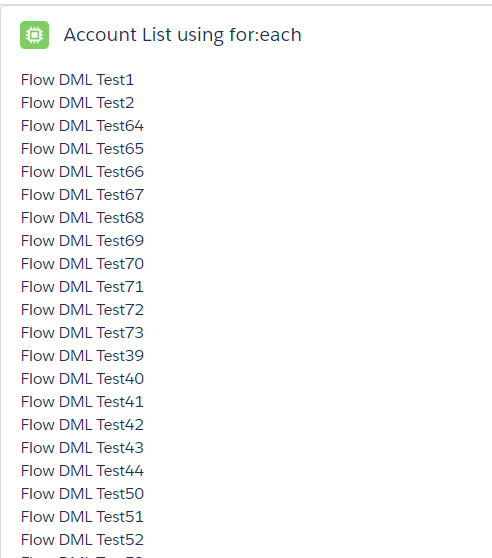
Output
iterating mechanism
For custom behavior applied to the initial or final item in a list, employ the iterator directive: iterator:iteratorName={array}. Implement this directive within a template tag.
Utilize iteratorName to access these attributes:
- value: Represents the item’s value within the list. Access array properties using this attribute, e.g., iteratorName.value.propertyName.
- index: Indicates the item’s index within the list.
- first: A boolean value signaling if this item is the first entry in the list.
- last: A boolean value signaling if this item is the final entry in the list.
Example: HelloIterator.html
<template> <lightning-card title="HelloIterator" icon-name="custom:custom14"> <ul class="slds-m-around_medium"> <template iterator:it={contacts}> <li key={it.value.Id}> <div if:true={it.first} class="list-first"></div> {it.value.Name}, {it.value.Title} <div if:true={it.last} class="list-last"></div> </li> </template> </ul> </lightning-card> </template>
helloIterator.css
.list-first { border-top: 1px solid black; padding-top: 5px; } .list-last { border-bottom: 1px solid black; padding-bottom: 5px; }
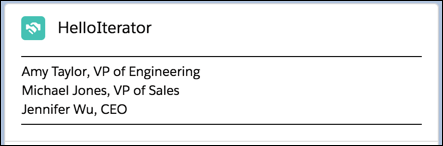