Salesforce Lightning Web Components (LWC) serves as Salesforce’s framework for effortless web application development. Imagine constructing innovative structures using building blocks—LWC embodies these blocks for websites and applications.
I’ve discovered an approach to seamlessly translate real-time information from Objects to the user interface. To accomplish this, I’ve outlined a three-step process. I’ll guide you through these steps in a beginner-friendly manner, simplifying the process of fetching and displaying data from Objects to the UI.
In this example, we’ll aim to retrieve feeds from the Custom Feed Object and showcase them on the UI.
The Three-step Guide entails:
Create Apex Controller
a. Apex Controller is like a middleman between Object (Salesforce’s Database) and LWC component. We have created a controller named FeedController.
b.Include a method (in our case getFeeds()) in order to fetch data.c. Don’t forget to add `@AuraEnabled(cacheable=true)` to enable Lightning component access and caching.
public with sharing class FeedController { @AuraEnabled(cacheable=true) public static List<Feed__c> getFeeds() { return [SELECT Id, Name, Post_Content__c, Post_Image__c, CreatedDate FROM Feed__c]; } }
Create LWC Component
a. Let’s create LWC component (feedPage in our case).
b.Import getFeeds method in our feedPage.js file.
import getFeeds from `@salesforce/apex/FeedController.getFeeds`;
c. Use @wire decorator to establish connection between Apex Controller and our component.
import { LightningElement, track, wire } from 'lwc'; import getFeeds from '@salesforce/apex/FeedController.getFeeds'; export default class FeedPage extends LightningElement { @track feeds; @wire(getFeeds) wiredFeeds({ error, data }) { if (data) { this.feeds = data; } else if (error) { console.error('Error fetching feeds:', error); } } }
Display on UI
With the data being fetched, now it’s time that we finally display it on our UI. This part will be handled by the feedPage.html file in our case. Show all feeds by using a for loop.
<template> <ul if:true={feeds}> <template for:each={feeds} for:item="dataItem"> <li key={dataItem.Id}> <!-- Display data item details here --> </li> </template> </ul> <p if:false={feeds}>No data found.</p> </template>
Important Admin configurations that are needed for your component to work properly on Builder of Digital Experience are listed below:
1. Enable Guest User Access.
2. Add a Sharing Rule in Sharing Settings.
3. Go to Profile < Settings < Builder and enable FeedController class.
4. Drag your component on the Builder’s Page.
5. Check your component, it will be fetching data and displaying the same.
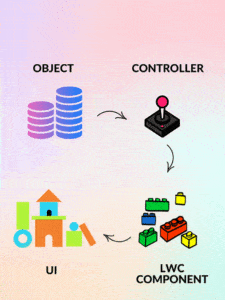
You have learned how to fetch and display data from Object to UI in Experience Cloud using Builder.
Closing Thoughts
This guide comprehensively covers concepts regarding retrieving and displaying data on UI webpages, featuring practical examples. In the given instance, we utilized a custom Feed Object (Feed___c) that includes various fields such as Name, Post_Content__c, Post_Image__c, Creator, among others. Our primary goal was to retrieve Feeds from this object and present them on our UI using an LWC Component.