Regarding Salesforce
Salesforce is a cloud-based CRM (Customer Relationship Management) solution that caters to various departments such as marketing, sales, commerce, and service. It offers a unified perspective of customers through a seamlessly integrated CRM platform.
Apex, Salesforce’s strongly typed, object-oriented programming language, empowers developers to execute flow and transaction control statements on Salesforce servers. Resembling Java syntax and functioning like database stored procedures, Apex allows the addition of business logic to numerous system events, including button clicks, related record updates, and Visualforce pages. Apex code can be triggered by web service requests and object triggers.
Within the realm of Apex, Collections serve as a variable capable of storing multiple records. The three main types of Collections are List, Set, and Map.
A Map, specifically, is a collection of key-value pairs where each unique key corresponds to a single value. Keys and values can encompass various data types, including primitive types, collections, sObjects, user-defined types, and built-in Apex types.
This article provides insights into understanding and testing Apex Map and its Methods in Salesforce.
For testing Apex Map in Salesforce, the prerequisites include:
- Registering for a Salesforce Free Trial account using the provided link.
Step 1
Log into your Salesforce account and click the “Developer Console”.
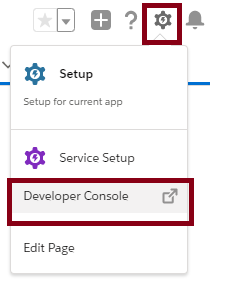
Step 2
The general syntax for Apex Map is,
Map<Key,Value> variablename=new Map<Key,Value>();
For learning more about Apex DataType, please refer to this link.
Now, we can create a new Apex Class named ApexMap and the file named as ApexMap.apxc.
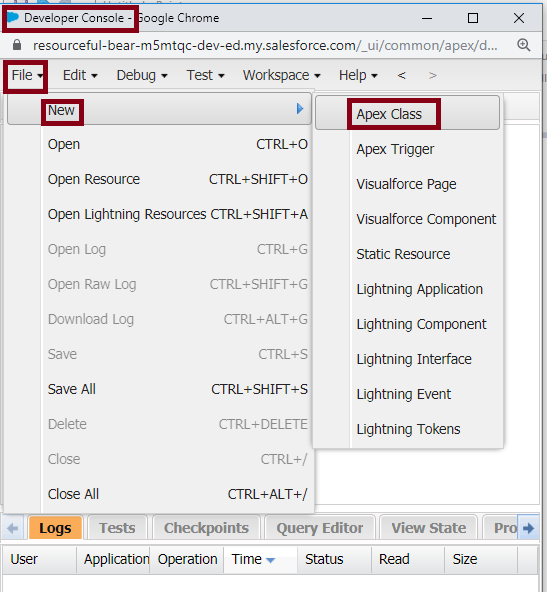
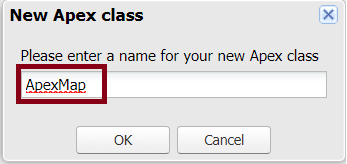
After creating the Apex class ApexMap, add a Maptest method for creating and testing the map and its methods.
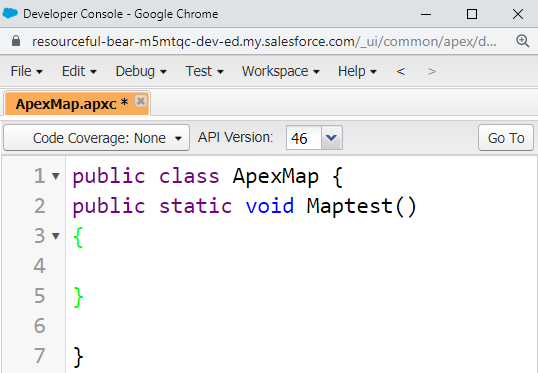
Step 3
Next, we can add some predefined important methods like put(key, value), get(key), keySet(), values(), size(), clone(), and clear() to the Apex Map.
put(key, value) – Associates the specified value with the specified key in the map. The code is,
Map<String, String> studDept=new Map<String, String>(); studDept.put('CSE','Kavin'); system.debug(studDept);
The get(key)
method allows retrieving the value associated with the key, or it returns null if the key is not mapped to any value.
keySet()- using this method, we can get the set of keys that contain in the map.
system.debug('Department keyset() are : '+ studDept.keyset());
values() – using this method, we can the set of values that contain in the map.
system.debug('Department values() are : '+ studDept.values());
size() – using this method, we can get the total number of key-value pairs in the Map.
Integer s=studDept.size(); system.debug('size() - Department Map Size : '+s);
clone() – using this method, we can get the duplicate copy of the map.
Map<String, String> studDeptc=studDept.clone(); system.debug('clone() - Department values() are : '+ studDeptc.values());
clear() – using this method, we can removes all the key-value mapping pairs from the map.
studDeptc.clear(); system.debug('clear() - Department values() are : '+ studDeptc.values());
Finally, the ApexMap.apxc class code is mentioned below.
public class ApexMap { public static void Maptest() { Map < String, String > studDept = new Map < String, String > (); //Put studDept.put('D01', 'CSE'); system.debug(studDept); //get(key) studDept.put('D02', 'CIVIL'); studDept.put('D03', 'MECH'); studDept.put('D04', 'EEE'); system.debug('get(D01) - Department is: ' + studDept.get('D01')); // keySet() system.debug('Department keyset() are : ' + studDept.keyset()); // values() system.debug('Department values() are : ' + studDept.values()); //Size() Integer s = studDept.size(); system.debug('size() - Department Map Size : ' + s); //clone() Map < String, String > studDeptc = studDept.clone(); system.debug('clone() - Department values() are : ' + studDeptc.values()); //clear studDeptc.clear(); system.debug('clear() - Department values() are : ' + studDeptc.values()); } }
Step 4
For debugging the Apex class ApexMap, click the Debug menu and select “Open Execute Anonymous Window”, as shown below.
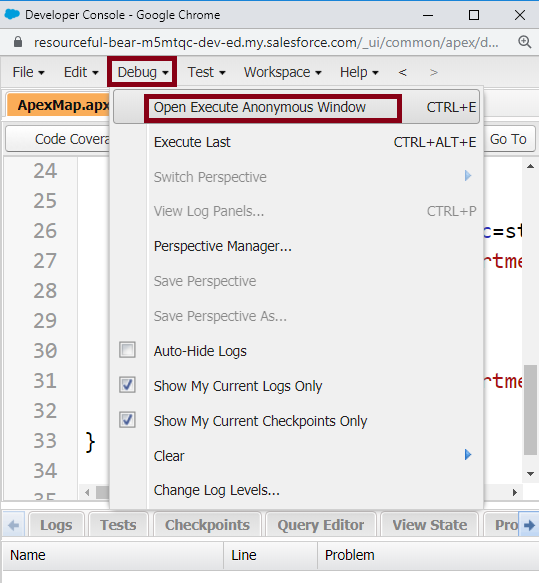
Now, write the Apex code for calling the Maptest() method, and enable the “Open log” option for viewing output and click “Execute”.
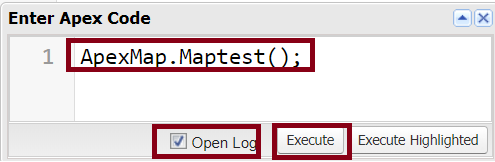
ApexMap.Maptest();
Step 4
Now, we can verify the output. After clicking Execute, the log will open automatically. Select “Debug Only”.
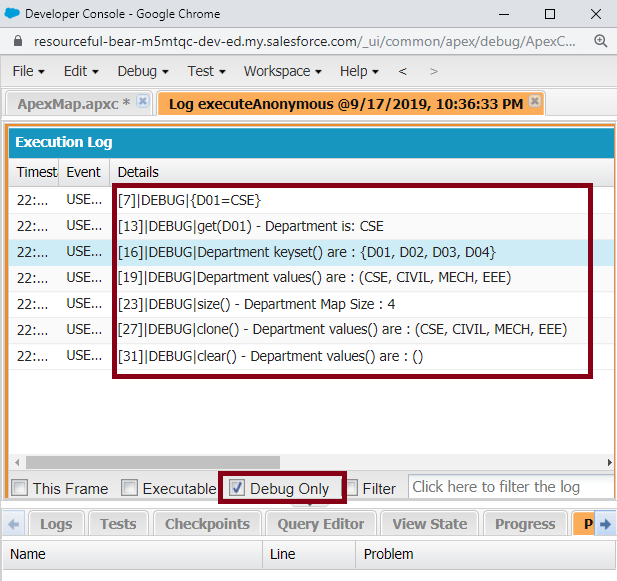
Synopsis
You have effectively conducted tests on Apex Map and its methods within the Salesforce environment.