Named and External Credentials are essential components for establishing secure data connections within Salesforce. Named Credentials act as references to external endpoints, allowing the platform to authenticate and access resources securely without exposing sensitive information like passwords.
On the other hand, External Credentials help enhance security by abstracting authentication details from the integration code. This separation of sensitive data from the codebase improves security measures. External Credentials can be utilized within Named Credentials to authenticate with external systems, offering a centralized and secure approach to managing authentication in integrations.
In this article, we’ll explore the process of adding authentication parameters to external credentials and accessing these parameters in Salesforce Apex Code. I’ve integrated Salesforce to utilize access tokens, which you can adapt according to your specific business needs.
Let’s delve into a step-by-step guide on how to utilize external credential parameters in your code:
- Set up a Salesforce Connect App
- Establish Named and External Credentials
- Develop Apex code to leverage External Credential Parameters
1. Establish a Salesforce Connect Application
Create a connected application within Salesforce. Follow steps 1 to 3 outlined in the “Generate Salesforce Authentication Token using Postman” post to set up a connected app.
2. Create Named and External Credential
External Credentials are used to store reusable authentication information for external systems. As we need to generate an access token for Salesforce, we need the below parameters,
client_id | Put the Consumer Key value from the connected app created from the above step. |
client_secret | Put the Consumer Secret value from the connected app created from the above step. |
username | Salesforce username that will be used to authenticate |
password | Salesforce username password + security token for the user. |
grant_type | password (hardcoded) |
Let us add these parameters to the external credential. Create an external credential Salesforce Login EC that will use a custom authentication protocol.
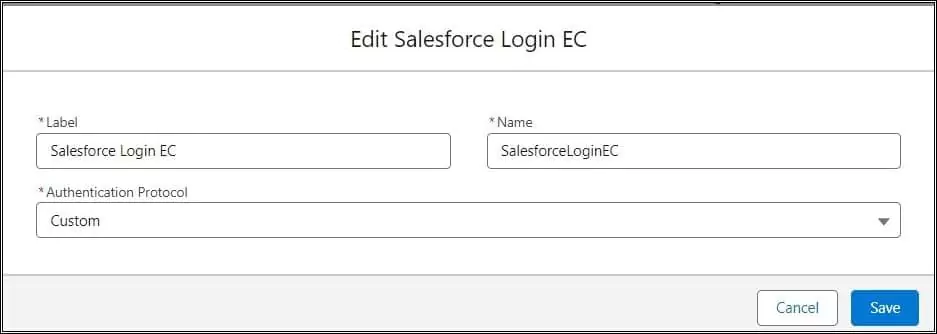
Create a Named Principal
A named principal applies authentication configuration to the named credential. We can use the same credential or authentication configuration for the entire org or we can use a per-user authentication configuration.
Let us create a named principal with the above-mentioned authentication parameters. Parameters added in the named principal are secure and its values are not visible to the user. Create a named credential SFLoginPrincipal.
Add all four types mentioned above in the named principal. In the below image, password and client_secret parameters are added. Add username and client_id as well.
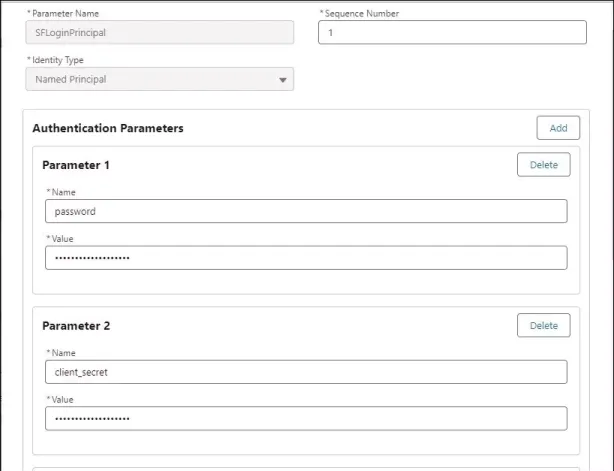
Establish a Named Credential.
Generate a named credential named “Salesforce Login NC” (SalesforceLoginNC) with the following properties.
URL | https://login.salesforce.com/services/oauth2/token |
Allow Callout | checked |
External Credential | Above created External Credential Salesforce Login EC |
Generate Authorization Header | Checked |
Allow Formulas in HTTP Header | Checked |
Allow Formulas in HTTP Body Help | Checked |
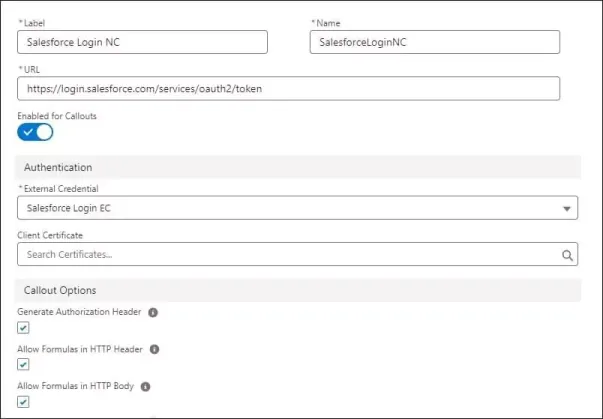
Named Credential in Salesforce
Create Permission Set
Create a permission set to provide user access. Create a permission set Salesforce Login PS and assign the above created external credential Salesforce Login EC in External Credential Principal Access. Once a permission set is created, assign this permission set to the required user. For this POC, I have added a permission set to myself.
3. Create Apex code to use External Credential Parameters
We have set up named and external credentials. Let us see how we can use the external credential parameters in Apex. To access external credential parameters, we have to access parameters like below
{!$Credential.<External Credential Name>.<Property Name>}. //Access client_id parameter {!$Credential.SalesforceLoginEC.client_id}
Let us use above-created parameters to get the Salesforce Access token using the apex class.
public class AccessTokenGenerator { private final static String REQUEST_BODY = 'grant_type=password&client_id={0}&client_secret={1}&username={2}&password={3}'; public static SFDCToken generateToken(string namedCredential) { SFDCToken token=null; HttpRequest request=new HttpRequest(); HttpResponse response=new HttpResponse(); Http httpRootObj = new Http(); request.setEndpoint('callout:'+namedCredential); request.setHeader('Content-Type','application/x-www-form-urlencoded'); request.setMethod('POST'); request.setBody(String.format(REQUEST_BODY,new string[]{'{!$Credential.SalesforceLoginEC.client_id}','{!$Credential.SalesforceLoginEC.client_secret}','{!$Credential.SalesforceLoginEC.username}','{!$Credential.SalesforceLoginEC.password}'})); request.setTimeout(60000); try { response= httpRootObj.send(request); token=SFDCToken.parse(response.getBody()); } catch(Exception expObj) { throw new BaseException(expObj); } return token; } }
public class BaseException extends Exception { }
4. Test Functionality
Let us test above created class by executing the command in the Developer Console.
//SalesforceLoginNC is Named credential name system.debug(JSON.serializePretty(AccessTokenGenerator.generateToken('SalesforceLoginNC')));
It will return token data as below
