The HTML specification underwent an update by the Web Hypertext Application Technology Working Group (WHATWG) in early 2021, eliminating support for the alert(), confirm(), and prompt() native APIs in third-party contexts. Consequently, browsers such as Chrome and Safari plan to discontinue support for the cross-origin usage of window.alert(), window.confirm(), and window.prompt() native APIs.
To resolve this issue within any LWC or Aura component, Salesforce has introduced LightningAlert, LightningConfirm, and LightningPrompt in the Summer ’22 release. Refer to the Summer ’22 release notes here for details.
To generate notifications from your Lightning web components, utilize the new modules LightningAlert, LightningConfirm, and LightningPrompt instead of relying on native APIs.
The following examples illustrate the usage of LightningAlert, LightningConfirm, and LightningPrompt within Lightning web components.
Illustration:
Let’s examine an example featuring three buttons within an LWC, each triggering different notifications: Alert, Confirm, and Prompt.
Here’s the code and the output showcasing the usage of LightningAlert, LightningConfirm, and LightningPrompt notifications.
<template> <lightning-card title="Notifications" icon-name="utility:user"> <div class="slds-box"> <lightning-button label="Alert" onclick={handleAlert}></lightning-button> <br /> <br /> <lightning-button label="Confirm" onclick={handleConfirm}></lightning-button> <br /> <br /> <lightning-button label="Prompt" onclick={handlePrompt} ></lightning-button> </div> </lightning-card> </template>
Within this demonstration, a modal is generated, comprising a header, a message, and two buttons. When the user inputs text and selects “OK” in the prompt, the .open() function triggers a promise that resolves to the entered value. Alternatively, if the user selects “Cancel,” the promise resolves to null.
import { LightningElement } from 'lwc'; import LightningAlert from "lightning/alert"; import LightningPrompt from "lightning/prompt"; import LightningConfirm from "lightning/confirm"; export default class Notification extends LightningElement { handleAlert() { LightningAlert.open({ message: "Alert Message", theme:"info", label: "Alert Header" }).then(() => { console.log("Alert Closed"); }); } handleConfirm() { LightningConfirm.open({ message: "Confirm Message", theme: "success", label: "Confirm Header" }); } handlePrompt() { LightningPrompt.open({ message: "Prompt Message", theme: "error", label: "Prompt Header", defaultValue: "Test" }).then(() => { console.log("Prompt closed"); }); } }
Result:
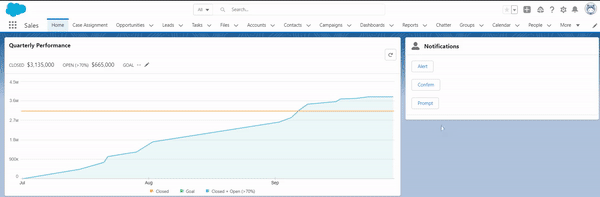