Custom Record search Functionality Using Lightning Web Component LWC
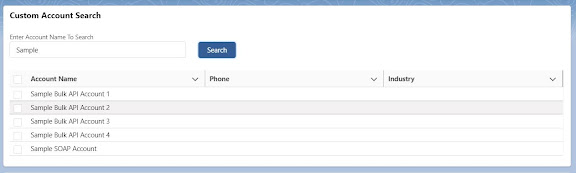
The Blog Post will show how to Create Custom Record Search Functionality on Account Object using Lightning Web Component i.e LWC
Please Follow the Below step.
Step 1:- Create Apex Class for Account search.
public class vlog_AccountSearch { @AuraEnabled(Cacheable = true) public static list<Account> getAccountData(String textkey) { textkey = '%' + textkey + '%'; list<Account> accLst = [SELECT Id, Name, Industry, Phone, Type From Account WHERE Name LIKE :textkey]; if(accLst.isEmpty()) { throw new AuraHandledException('No Record Found..'); } return accLst; } }
Step 2:- Create HTML component for Account search.
<template> <lightning-card title="Custom Account Search"> <lightning-layout multiple-rows="true" vertical-align="end"> <lightning-layout-item size="4" padding="around-small"> <!--Input text to Search Account--> <lightning-input type="text" label="Enter Account Name To Search" value={searchKey} onchange={handelSearchKey}> </lightning-input> </lightning-layout-item > <lightning-layout-item size="2" padding="around-small"> <!--Search Button--> <lightning-button label="Search" variant="brand" onclick={SearchAccountHandler}> </lightning-button> </lightning-layout-item> <lightning-layout-item size="12" padding="around-small"> <!--List Of Account Using data Tabel--> <lightning-datatable key-field="id" data={accounts} columns ={cols}> </lightning-datatable> </lightning-layout-item> </lightning-layout> </lightning-card> </template>
Step 3:- Create a Javascript controller for Account search.
import { LightningElement, track } from 'lwc'; import getAccountData from '@salesforce/apex/vlog_AccountSearch.getAccountData'; export default class CustomRecordSearch extends LightningElement { searchKey; @track accounts; //This Funcation will get the value from Text Input. handelSearchKey(event){ this.searchKey = event.target.value; } //This funcation will fetch the Account Name on basis of searchkey SearchAccountHandler(){ //call Apex method. getAccountData({textkey: this.searchKey}) .then(result => { this.accounts = result; }) .catch( error=>{ this.accounts = null; }); } cols = [ {label:'Account Name', fieldName:'Name' , type:'text'} , {label:'Phone', fieldName:'Phone' , type:'Phone'} , {label:'Industry', fieldName:'Industry' , type:'text'} ] }
Step 4:- Create Meta.xml for visibility of web component.
<?xml version="1.0" encoding="UTF-8"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata"> <apiVersion>52.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__RecordPage</target> <target>lightning__AppPage</target> <target>lightningCommunity__Page</target> <target>lightningCommunity__Default</target> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>