In this section, we’ll delve into the errorCallback() function in Lightning Web Component.
- It captures errors that occur within all child components’ lifecycle hooks.
- This method is exclusive to LWC.
- It takes two parameters: error and stack. The error parameter represents a JavaScript native error object, while the stack parameter is a string.
- Errors occurring in the parent component display in the UI without a boundary component. This implies that if an error arises in a child component, the parent handles it. However, if the error originates in the parent, it’s showcased in the UI.
- This method operates akin to a JavaScript catch() block, serving to catch and manage errors.
Sample
errorCallback(error, stack){ console.log('errorcallback - grandparent' + error ); console.log(stack); }
Example
parent.html
<template> I am in parent comonent<br/> <c-child> </c-child> </template>
parent.js
import { LightningElement } from 'lwc'; export default class App extends LightningElement { errorCallback(error, stack){ console.log('errorcallback -parent' + error ); console.log(stack); } }
child.html
<template> I am in child component </template>
child.js
import { LightningElement} from 'lwc'; export default class App extends LightningElement { constructor(){ super(); console.log('constructor - child'); throw 'woops error' } errorCallback(error, stack){ console.log('errorcallback - child' + error ); console.log(stack); } }
Console Output
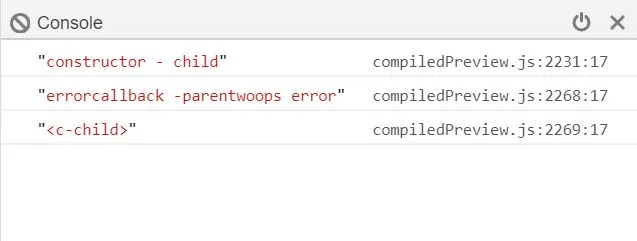
In the console, we observe that the error is managed by the parent component due to the presence of the errorCallback() within it.
Had we not included errorCallback() in the parent component, the output would appear as follows:
Additionally, it’s important to highlight that while errors from the child component are managed by the parent, errors originating in the parent component will be visible in the UI.